When it comes to solving problems in programming, recursion and iteration are two fundamental techniques that often achieve the same result but through very different approaches. Understanding when and how to use each can make a significant difference in the efficiency, readability, and maintainability of your code.
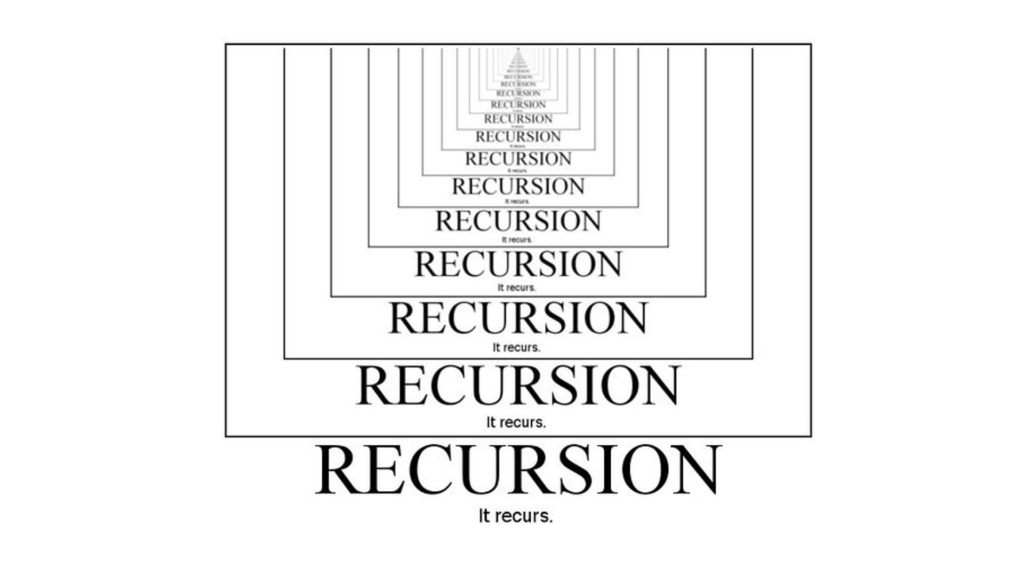
What is Recursion?
Recursion occurs when a function calls itself to solve smaller instances of the same problem until it reaches a base case, at which point the function stops calling itself. It’s like solving a puzzle by breaking it down into smaller pieces, solving each piece, and then combining the solutions.
Example: Calculating Factorials
A classic example of recursion is calculating the factorial of a number n
, which is the product of all positive integers less than or equal to n
.
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n - 1)
# Example usage
print(factorial(5)) # Output: 120
In this example, factorial(5)
calls factorial(4)
, which calls factorial(3)
, and so on, until it reaches the base case of factorial(1)
.
What is Iteration?
Iteration, on the other hand, involves using loops to repeatedly execute a block of code until a certain condition is met. This approach is more linear and straightforward, processing one step at a time.
Example: Calculating Factorials with a Loop
The same factorial problem can be solved using iteration with a loop:
def factorial(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
# Example usage
print(factorial(5)) # Output: 120
Here, the loop runs from 1
to n
, multiplying each number by the running total, and producing the same result as the recursive version.
When to Use Recursion
Recursion is particularly useful when a problem can naturally be divided into similar subproblems. Some common scenarios include:
- Tree Traversal: Navigating tree structures, such as parsing expressions or organizing hierarchical data.
- Divide and Conquer Algorithms: Problems like merge sort or quicksort, where the problem is divided into smaller, independent subproblems.
- Backtracking: Exploring all possible configurations, such as solving puzzles like Sudoku or finding all possible paths in a maze.
Advantages:
- Simplicity: Recursive solutions are often more intuitive and easier to write, especially for problems that naturally fit a recursive structure.
- Elegant Code: Recursion can lead to cleaner, more readable code when used appropriately.
Disadvantages:
- Performance: Recursion can be less efficient due to the overhead of multiple function calls and increased memory usage from the call stack.
- Risk of Stack Overflow: If the recursion depth is too deep, it can lead to a stack overflow error.
When to Use Iteration
Iteration is generally preferred when the problem involves repetitive tasks that don’t naturally break down into subproblems. It’s often used in:
- Simple Loops: Tasks like summing elements in an array, counting occurrences, or iterating through collections.
- Performance-Critical Code: Iteration is usually more efficient in terms of memory and speed, making it ideal for performance-sensitive applications.
- Finite Processes: When you know exactly how many times a loop should run, iteration is a straightforward and efficient approach.
Advantages:
- Efficiency: Iteration avoids the overhead of function calls, making it faster and more memory-efficient.
- Control: It provides precise control over the looping process, allowing for easy implementation of complex logic.
Disadvantages:
- Complexity: Iterative solutions can sometimes be more complex and harder to read, especially for problems that naturally lend themselves to recursion.
- Verbose Code: Iterative solutions can become cumbersome and lengthy, reducing code readability.
How to Choose Between Recursion and Iteration
The choice between recursion and iteration often comes down to the nature of the problem and your priorities:
- Use Recursion When:
- The problem is naturally recursive (e.g., tree traversal).
- You prefer cleaner, more readable code and are not concerned about performance.
- The recursive depth is manageable, and the risk of stack overflow is low.
- Use Iteration When:
- Performance is a critical concern, and you need to minimize memory usage.
- The problem involves simple repetition or iteration over a collection.
- You need precise control over the looping process or expect a large number of iterations.
Conclusion
Both recursion and iteration are valuable tools in a programmer’s toolkit, each with its own strengths and weaknesses. By understanding the nature of the problem you’re solving and the trade-offs of each approach, you can choose the technique that best suits your needs. Whether you opt for the elegance of recursion or the efficiency of iteration, mastering both will make you a more versatile and effective coder.