In the world of algorithmic problem-solving, the two-pointer technique is a simple yet incredibly effective tool that can make your solutions more efficient. Whether you’re working on arrays, linked lists, or strings, this approach can help you tackle complex problems with ease.
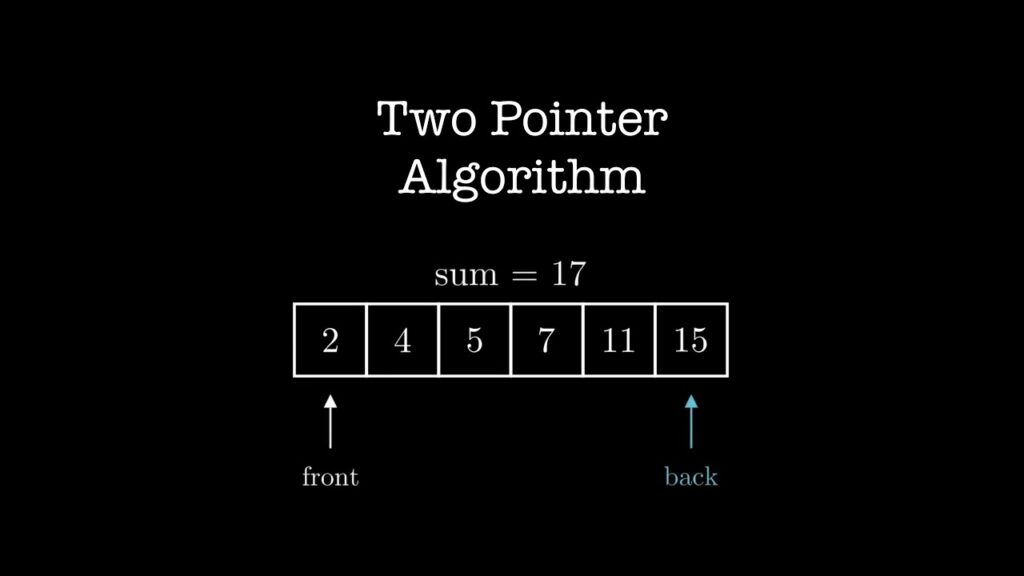
What is the Two Pointers Technique?
The two-pointer technique involves using two indices (or “pointers”) to traverse a data structure, typically from different directions, to solve a problem. By strategically moving these pointers, you can reduce the need for nested loops and, in many cases, bring down the time complexity of your solution from O(n^2) to O(n).
How Does It Work?
Imagine you have a sorted array, and you want to find a pair of numbers that add up to a specific target. Instead of using a brute force approach (checking every possible pair), you can use the two pointers technique:
- Initialize: Start with one pointer at the beginning of the array (
left = 0
) and the other at the end (right = n-1
). - Check the Sum: Add the values at these pointers:
- If the sum equals the target, you’ve found your pair.
- If the sum is less than the target, move the left pointer one step to the right (
left++
). - If the sum is greater than the target, move the right pointer one step to the left (
right--
).
- Continue: Repeat until the pointers meet or you find the pair.
This simple strategy allows you to find the solution in linear time.
Common Use Cases
- Pair Sum in a Sorted Array: As discussed, find two numbers that add up to a target.
- Reversing a String: Use two pointers starting at the beginning and end of the string, swapping characters until they meet in the middle.
- Merging Two Sorted Arrays: Move pointers through both arrays to merge them in sorted order.
Example Code: Pair Sum
Here’s a quick Python example of finding a pair in a sorted array that sums to a target:
def find_pair(arr, target):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return (arr[left], arr[right])
elif current_sum < target:
left += 1
else:
right -= 1
return None
# Example usage
arr = [1, 2, 3, 4, 6]
target = 8
print(find_pair(arr, target)) # Output: (2, 6)
Why Use the Two Pointers Technique?
- Efficiency: Reduces unnecessary computations, making your algorithms faster.
- Simplicity: Easy to implement and understand, yet powerful in its application.
- Versatility: Applicable to a wide range of problems, especially those involving sorted data or symmetrical operations.
Conclusion
The two-pointers technique is a must-know tool in your algorithmic toolkit. It provides a clear path to more efficient solutions for common problems, making it easier to write clean, optimized code. Whether you’re preparing for coding interviews or looking to improve your problem-solving skills, mastering this technique will undoubtedly give you an edge.
Happy coding!